Multi-Echo FLASH Tutorial#
This tutorial extends the FLASH Tutorial to show a more complex readout pattern with alternating polarities and how to add other matplotlib objects to a sequence diagram.
We will start from a simple diagram with only three channels, the RF pulses, the readout gradient and the echoes.
import copy
import matplotlib.pyplot
import mrsd
import numpy
figure, plot = matplotlib.pyplot.subplots(tight_layout=True)
diagram = mrsd.Diagram(plot, ["RF", "$G_{RO}$", "Echoes"])
We will then define sequence parameters, add the RF pulse and show the TR. Note that this diagram uses a rectangular pulse instead of a sinc one.
T2_star = 5
TE, TR = 4, 20
d_pulse, d_readout, d_ramp = 0.5, 1, 0.1
train_length = 10
pulse = diagram.hard_pulse("RF", d_pulse, 1, center=0)
diagram.add("RF", copy.copy(pulse).move(TR))
diagram.interval(0, TR, -1.5, "TR")
We then add the first echo, its readout gradient and the prephasing lobe of the readout gradient. In this example, we do not show the ADC to make the diagram more readable.
echo = diagram.echo("Echoes", d_readout, 1, center=TE)
readout = diagram.gradient("$G_{RO}$", d_readout, 1, d_ramp, center=echo.center)
diagram.add(
"$G_{RO}$", readout.adapt(d_readout, -0.5, d_ramp, end=readout.begin))
This part is very similar to the FLASH Tutorial and yields the following diagram.
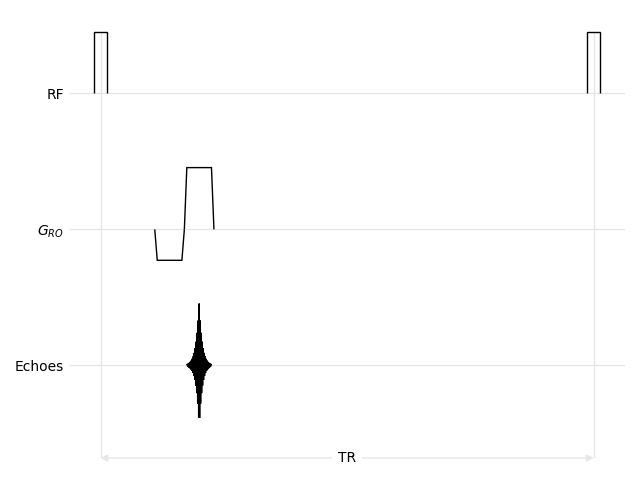
Single echo#
Multiple Echoes#
In this tutorial, the sequence uses bipolar readout gradients and echoes as close as possible with respect to the gradient ramps. This means that the beginning of a readout gradient is the end of a previous one, and that the center of each echo is located at the center of its corresponding gradient lobe. We also modulate the echo amplitudes with a \(T_2^*\) decay.
for echo in range(1, train_length):
gradient_amplitude = (-1)**echo
readout = diagram.gradient(
"$G_{RO}$", d_readout, gradient_amplitude, ramp=d_ramp,
begin=readout.end)
elapsed = readout.center - TE
echo_amplitude = numpy.exp(-elapsed/T2_star)
diagram.echo("Echoes", d_readout, echo_amplitude, center=readout.center)
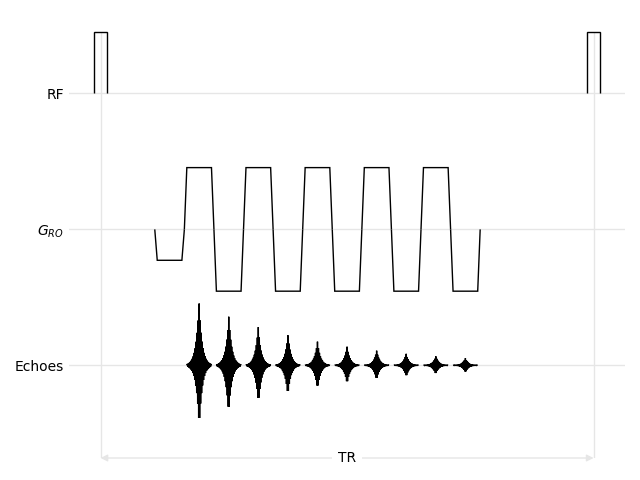
Multiple echoes#
T₂* Decay#
Finally, we show a continuous \(T_2^*\) decay curve overlayed on top of the echoes. This is achieved by calling the usual matplotlib functions of the plot
object, with the curve beginning at the first echo located at TE
and ending at the last echo, using the last readout
object. The vertical position must set to the RF channel, which can be accessed using the y()
function of the Diagram
class.
xs = numpy.linspace(0, readout.end-TE, 100)
ys = numpy.exp(-xs/T2_star)
plot.plot(xs+TE, ys+diagram.y("Echoes"), color="C0", lw=1)
plot.text(TE+xs[len(xs)//2], 0.5, "$e^{-t/T_2^*}$", color="C0")
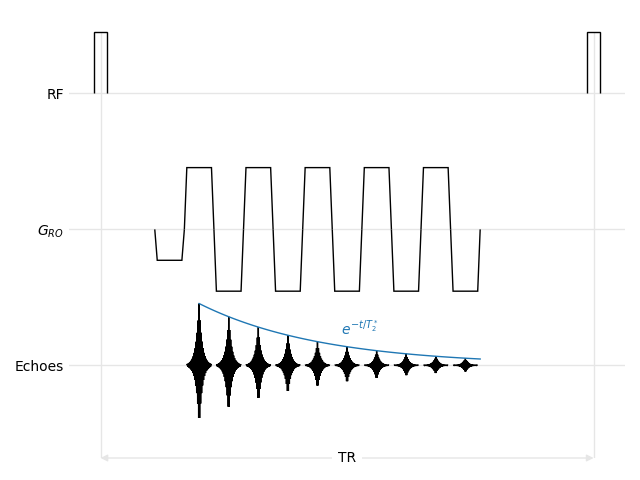
Multiple echoes and overlayed T₂* decay#